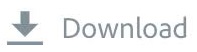

This makes the code less prone to error, as the syntax is simplified. On the other hand, key performs the computation only once. When cmp is passed as a parameter, the sorting algorithm compares pairs of values and the comparing function is called multiple times for every item. In Python 3, the cmp parameter has been removed, mainly for two reasons.įirst, everything done with cmp can be done with key. > sorted_l = sorted(l, key=lambda x: x ** 3) # Sort with key > sorted_l = sorted(l, cmp=lambda x, y: x ** 3 - y ** 3) # Sort with cmp > # The cmp parameter has been removed in Python 3
#Python 3 sort list stack overflow how to#
Knowing how to manipulate data, write custom sort functions in Python, and perform custom comparisons are essential skills to master. You can learn more about custom objects in Python in the article Simple Steps for Creating Your Own Class in Python. > sorted(pokemon_objects, key=lambda x: x.attack) # sort by attack return repr((self.name, self.category, self.attack)) def _init_(self, name, category, attack): It also works for objects with name attributes: > sorted(pokemon, key=lambda x: x) # sort by attack power For example, we can define a custom order to sort a list of tuples: Because the key function is called only once for each input record, this is an efficient way to perform sorting in Python.Ī common pattern is to sort complex objects using some of the object’s indices as key. The value of the key parameter should be a function that takes a single argument and returns a key for sorting purposes. In Python, we can write custom sort functions that work with sort() and sorted(). Now, let’s talk about custom sort functions in Python.

Note: It is common to pass a custom lambda function as a key parameter to sort complex objects in Python. > sorted(" is awesome to learn about custom sort functions in Python".split(), key=str.lower) Last but not least, list.sort() can only work on lists while sorted() accepts any iterable.įor example, here’s a case-insensitive string comparison: It is also important to note that sorted() will return a list therefore, you have to assign the output to a new variable.Īs for list.sort(), it modifies the list in place and has no return value.

However, this comes at the cost of convenience. Because sort() does not make any copy of the initial variable, it is a bit more efficient than sorted(). On the other hand, sorted() keeps a copy of the initial variable, making it possible to revert to the initial order if needed. One important thing to note is that sort() modifies the initial variable directly, and consequently, the initial order will be lost. In terms of syntax, sort() is an instance method implemented as list_to_sort.sort(), while sorted() is used as sorted(list_to_sort). Sorting With Custom Sort Function in Pythonįirst, let’s talk about the difference between sort() and sorted(). If you are not comfortable with Python functions, it is a good idea to read How to Define a Function in Python before diving deeper into this article. In other words, I’ll explain how to use a custom lambda function as a key parameter. In this article, I want to go further on the sort topic and explore how to write a custom sort function in Python.

Both list.sort() and sorted() have a key parameter that specifies a function to be called on each list element before making comparisons. In my previous article on working with streams in Python, I briefly introduced sorting methods with list.sort() and sorted(). Let’s discover how to use custom sort functions to implement custom orders and comparisons in Python. They are important because they often reduce the complexity of a problem. In computer science, a sorting algorithm puts elements of a list into a particular order.
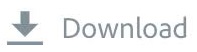